Aave Liquidity Provision
Background
Aave is a decentralized liquidity protocol that allows users to lend and borrow a variety of cryptocurrencies in a trustless and non-custodial manner. Aave is deployed on several networks such as Ethereum, Arbitrum, Polygon and more.
Users can deposit their digital assets into liquidity pools, making them available for others to borrow. In return, depositors earn interest on their assets, which is dynamically calculated based on supply and demand metrics.
Borrowers on Aave can take out loans by providing collateral in the form of other assets. This ensures the security and stability of the protocol.
Aave is known for its support for flash loans, which are uncollaterized loans that must be repaid within the same block. This enables users to execute complex trading strategies without needing upfront capital.
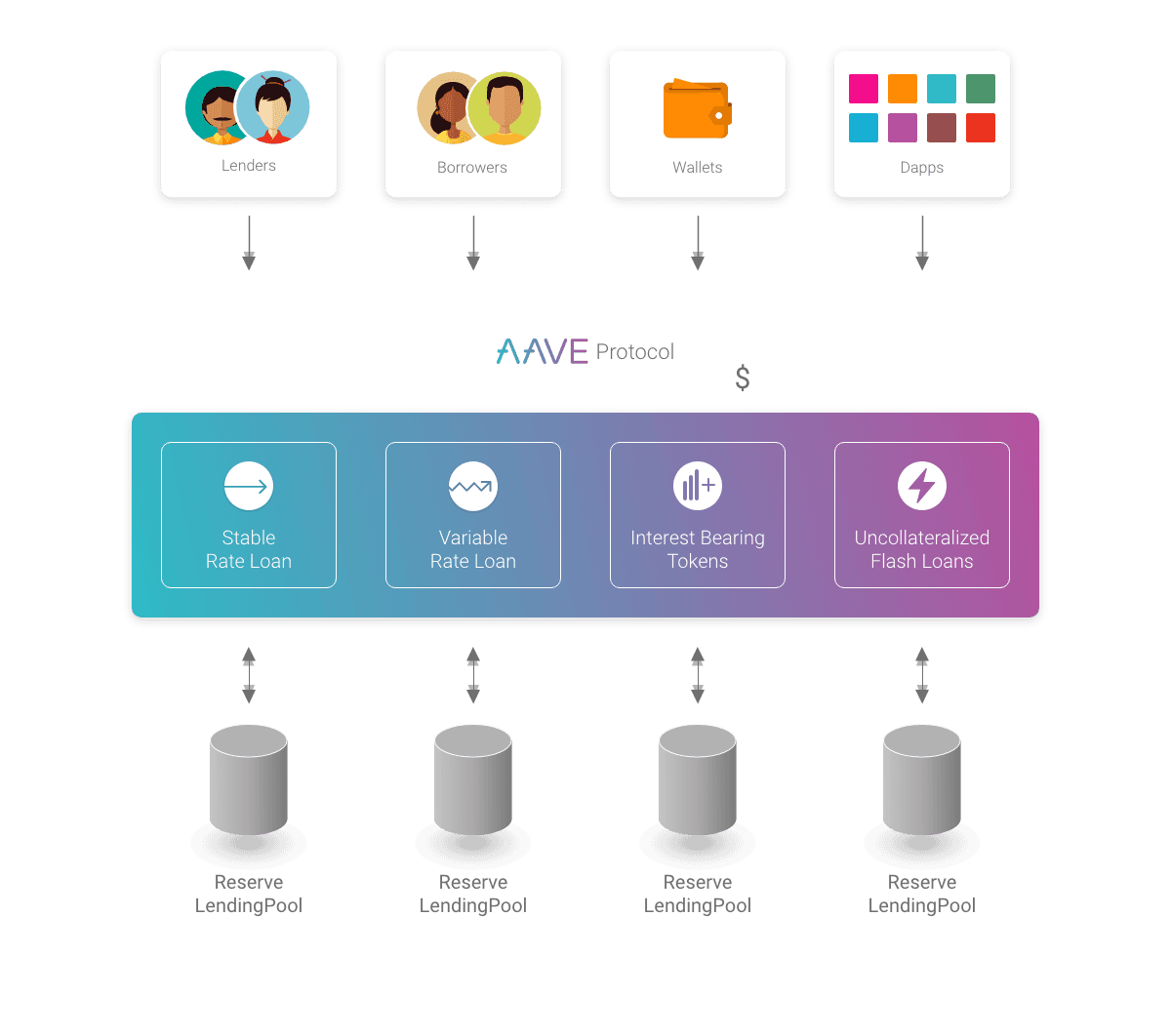
Passive Liquidity Provision
The agent only supplies liquidity at the start of the simulation. We set self.has_invested
to False
initially and change it to True
after we supply liquidity.
Afterwards, we track the health factor of the agent. health_factor
is calculated and given to us by the Aave smart contract.
Aave's health factor is a metric that determines a user's loan-to-value ratio and indicates how safe their funds are from liquidation.
So a higher health factor means the agent's collateral is more than enough to cover its loan. A health factor of 1 triggers Aave to liquidate your assets.
In our policy, we try to keep health factor between 1.7 and 2 by borrowing when it's above 2 and repaying when it's below 1.7.
How To Run
Installation
Follow our Getting Started guide to install the dojo library and other required tools.
Then clone the dojo_examples
repository and go into the relevant directory.
git clone https://github.com/CompassLabs/dojo_examples.git
cd dojo_examples/examples/aavev3
Running
Download the dashboard to view the simulation results.
To view example simulation data, download aavev3.db
from here and click 'Add A Simulation' on the dashboard.
To run the simulation yourself, use the following command.
python run.py
This command will setup your local blockchain, contracts, accounts and agents. You can then access your results on your Dojo dashboard by connecting to a running simulation.
Step-By-Step Explanation
Initialization
We create a class called AAVEv3Policy
which inherits from the BasePolicy
class and initializes the self.has_invested
variable which will be used later on.
class AAVEv3Policy(BaseAAVEv3Policy):
"""Provide liquidity passively to a pool in the specified price bounds."""
def __init__(self) -> None:
"""Initialize the policy."""
super().__init__()
self.has_invested = False
Supplying Liquidity
In this policy, we would like to provide liquidity to the pool passively. Therefore, we return a AaveV3Supply
object with the relevant parameters set only the first time we run this simulation.
def predict(self, obs: AAVEv3Observation) -> list[BaseAaveAction]:
"""Derive actions from observations."""
if not self.has_invested:
self.has_invested = True
return [
AAVEv3Supply(agent=self.agent, token="USDC", amount=Decimal("30000"))
]
Risk Management
In this policy, the agent tries to keep the health factor between 1.7 and 2.
If the health factor is greater than 2, the agent borrows enough WBTC tokens to bring the health factor down to 1.7. This is because borrowing an asset increases the agent's debt.
If the health factor is less than 1.7, the agent repays enough WBTC tokens to bring the health factor up to 2. This is because repaying an asset reduces the agent's debt, making the position safer.
health_factor = obs.get_user_account_data_base(
self.agent.original_address
).healthFactor
if health_factor > 2.0:
return [
AAVEv3BorrowToHealthFactor(
agent=self.agent,
token="WBTC",
factor=1.8,
mode=BorrowingMode.VARIABLE,
)
]
if health_factor < 1.7:
return [
AAVEv3RepayToHealthFactor(
agent=self.agent,
token="WBTC",
factor=1.90,
mode=BorrowingMode.VARIABLE,
)
]
return []
In the run.py
file, we create an Aave environment and an agent that implements the AaveV3 policy.
Results
You can download the results to this example below.
We offer a dashboard desktop application for visualizing your simulation results. You can download the file for the desktop application here, or just open the results in our hosted dashboard.